How to create a PDF document with C#
Sooner or later you’ll face the need to create a PDF document programmatically, from your C# code. It is totally possible with the help of iTextSharp library.
iTextSharp is available via NuGet, so just add it in you project:
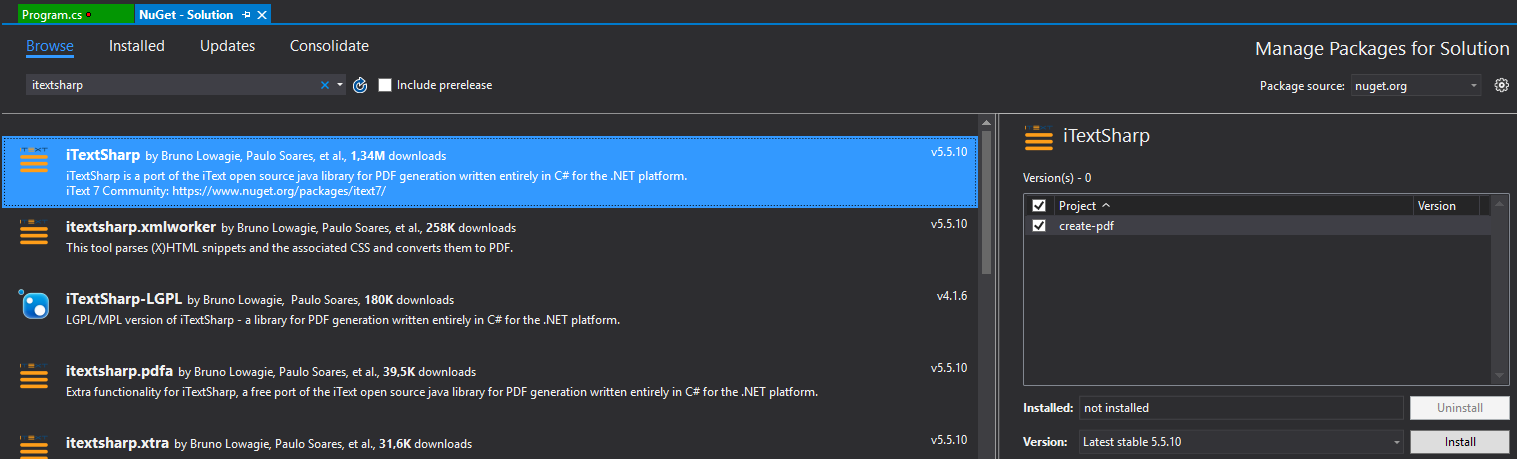
And now you can use it like this:
- Prepare some font objects, like one for normal paragraphs, one for bold ones, one for headers and so on;
- Create a document object;
- Fill it with some content.
Here’s how you can define a font:
string normal_font = Path.Combine(
Environment.GetFolderPath(Environment.SpecialFolder.Fonts),
"verdana.ttf" // that means, that we will use Verdana font
// look for more fonts in your system
);
FontFactory.Register(normal_font);
BaseFont baseFont_normal = BaseFont.CreateFont(
normal_font,
BaseFont.IDENTITY_H,
BaseFont.NOT_EMBEDDED
);
Font font_main = new Font(baseFont_normal, 10, Font.NORMAL);
Now we have font_main
object that can be used in our document.
Document is created like this:
using (FileStream fs = new FileStream(PATH-TO-THE-FILE, FileMode.Create, FileAccess.Write, FileShare.None))
using (Document doc = new Document(PageSize.A4, 80, 80, 70, 70)) // some layout settings
using (PdfWriter writer = PdfWriter.GetInstance(doc, fs))
{
writer.ViewerPreferences = PdfWriter.HideMenubar;
doc.Open();
// document's content goes here
doc.Close();
}
Filling it with content is being done by simple statements like:
doc.Add(new Paragraph("Some text", font_main));
Note, how we’re using font_main
for that paragraph.
Paragraphs can have different alignment, so it might be useful to create a function for that:
static Paragraph createAlignedParagraph(string text, Font font, int alignment)
{
Paragraph p = new Paragraph(text, font);
p.Alignment = alignment;
return p;
}
And now we can create center-aligned paragraph like this:
doc.Add(createAlignedParagraph("Some text", font_main, Element.ALIGN_CENTER));
You can also fill some meta-information:
doc.AddTitle("Attack on Numbani");
doc.AddSubject("Match results");
doc.AddKeywords("pdf, example");
doc.AddCreator("Overwatch");
doc.AddAuthor("Athena");
Which will be stored in the document properties:
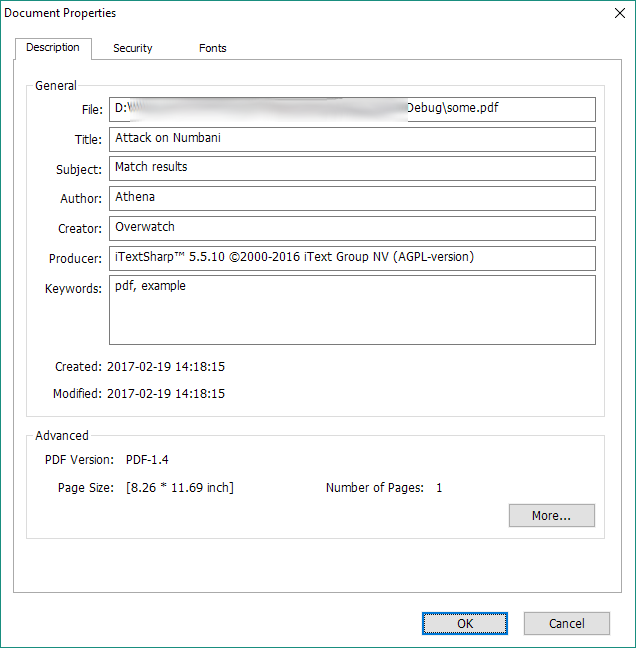
To make it easier for you, I’ve created a simple console application that produces the following PDF document:
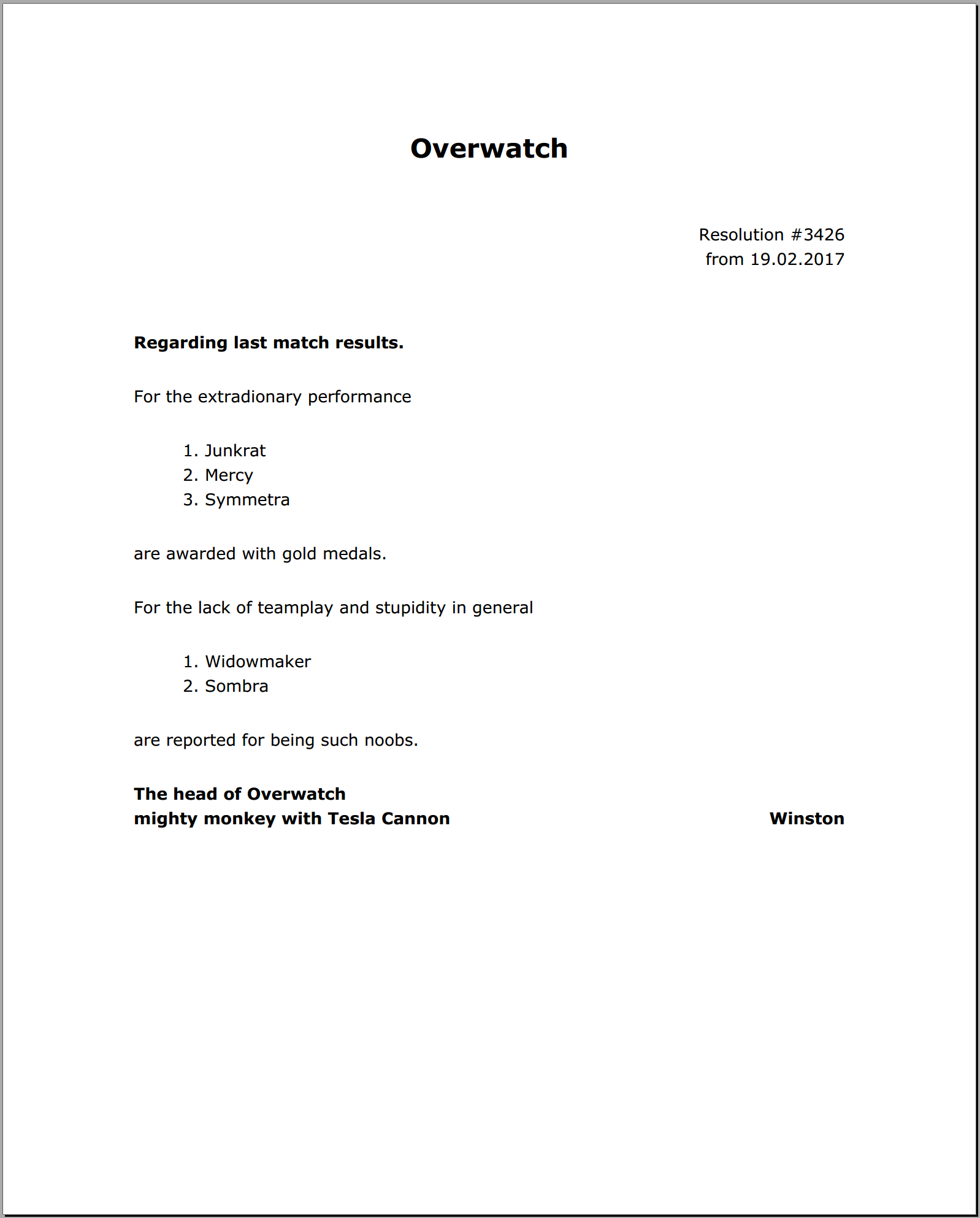
The source code of the application is available here.
Social networks
Zuck: Just ask
Zuck: I have over 4,000 emails, pictures, addresses, SNS
smb: What? How'd you manage that one?
Zuck: People just submitted it.
Zuck: I don't know why.
Zuck: They "trust me"
Zuck: Dumb fucks